AssociatedType in Swift – A Generic Adventure
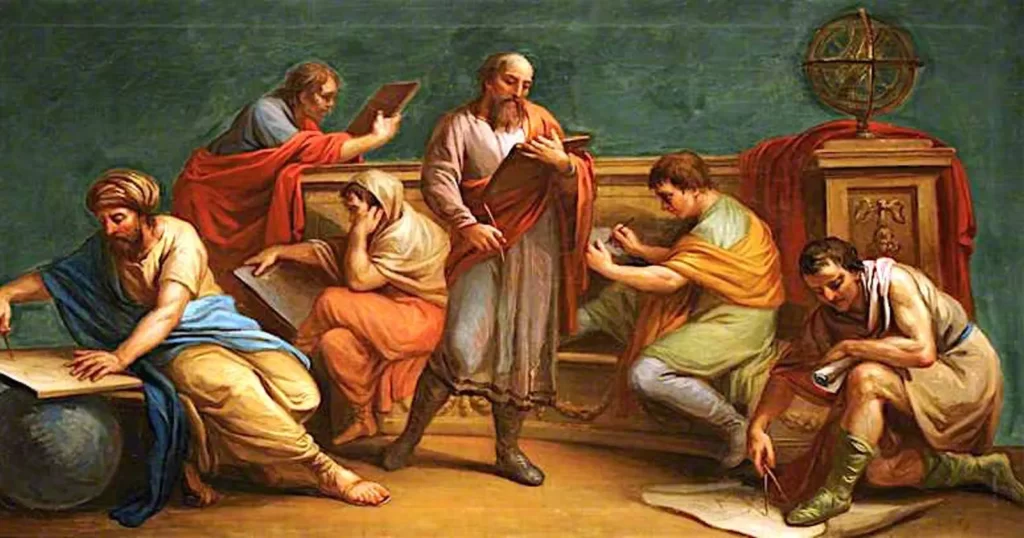
Hello ladies and gentlemen, Leo here. Today we’ll explore a great language feature called associatedtype in Swift. In general terms, associatedtype is used when you need to have generic behavior in your protocols. It’s important because as Apple says: Generic code enables you to write flexible, reusable functions and types that can work with any type, […]
Algorithms – Longest Increasing Subsequence in Swift
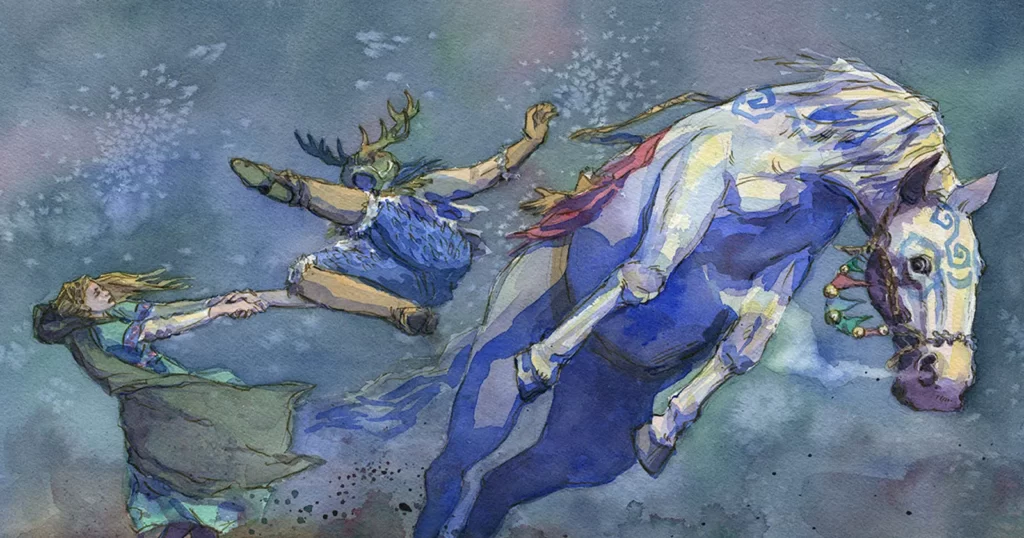
Hello everyone, Leo here. Today we’ll explore one of the Longest Increasing Subsequence in Swift, also known as LIS problems. The LIS is a very classic problem of computer science and has various approaches. Today we’ll examine the Dynamic Programming approach because it is very straighforward. Remember the dynamic programming solution is NOT the optimal solution, […]
Copy-on-write in Swift
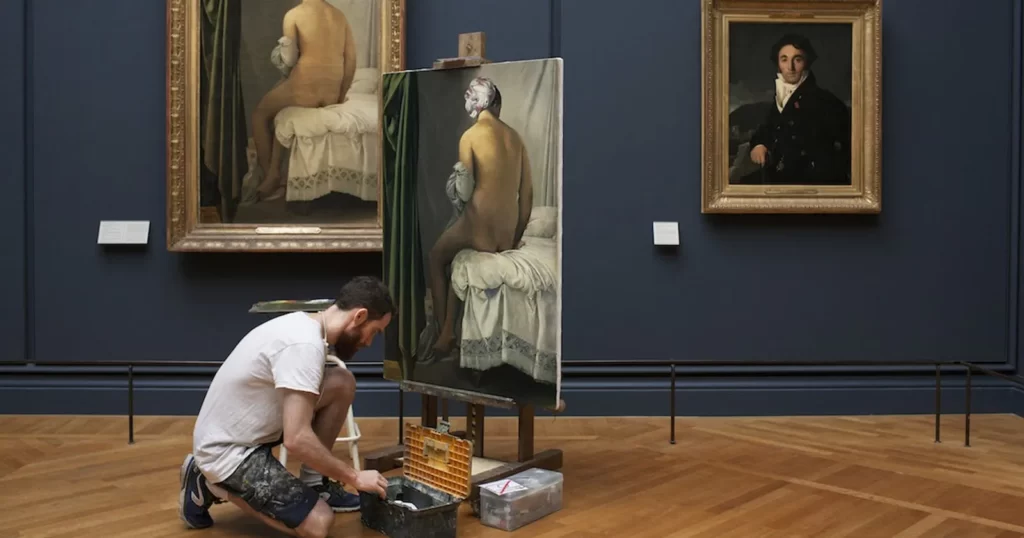
Hello ladies and gentlemen, Leo here. The day has come and we will talk about Copy-on-write in Swift. Yes, this is one of the most important features of the Swift programming language and all iOS developers should know what and how this happens under the hood. First, we will introduce the differences between value types […]
Using Cryptography Asymmetric Keys in Swift
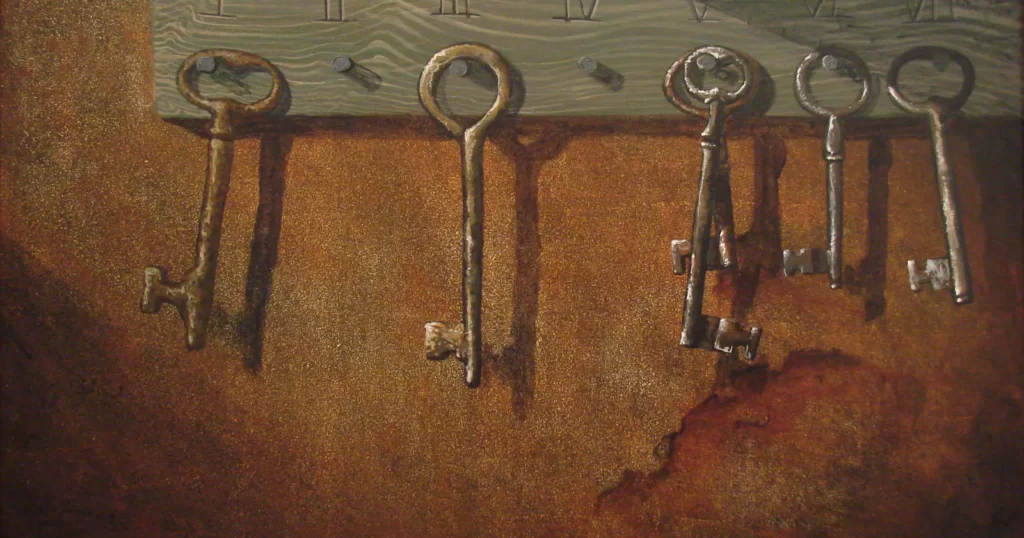
Hello, fellow tech developers, Leo here. Do you ever consider studying or using Cryptography Asymmetric Keys in Swift? My hope is that this article helps you on solving your cryptography problems. Having cryptographic problems is not easy, so let’s try to make that a little bit more intuitive today. This week we’ll explore some old […]
Expression Pattern Operator in Swift
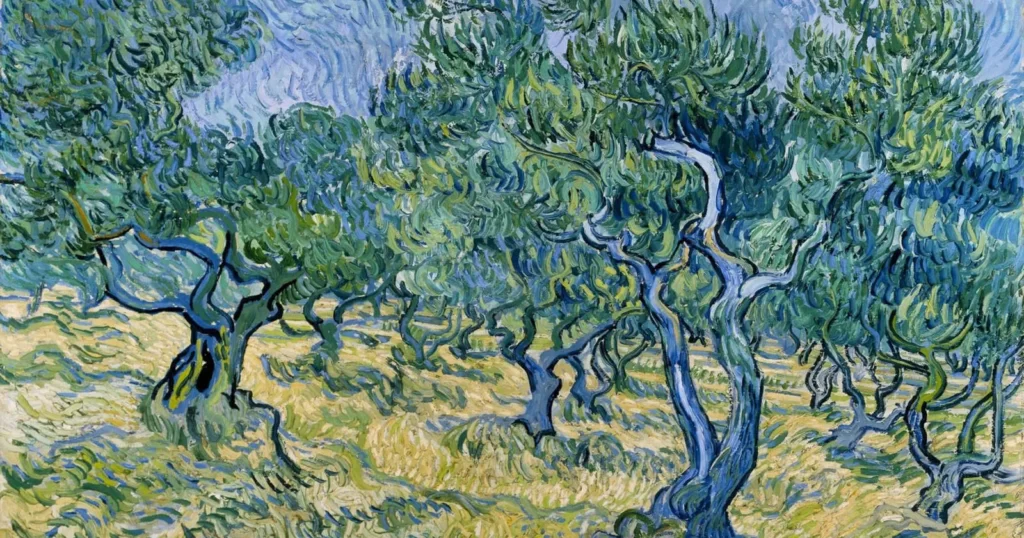
Hello people, Leo here. I’m cheerful to say that today we’ll study the Expression Pattern Operator in Swift. We’ll explore one kind of hidden operator called pattern matching operator or expression pattern operator. This is behind the scene and used as a *switch* statement operator by Swift. So let’s see how can we use it […]
OperationQueue in Swift
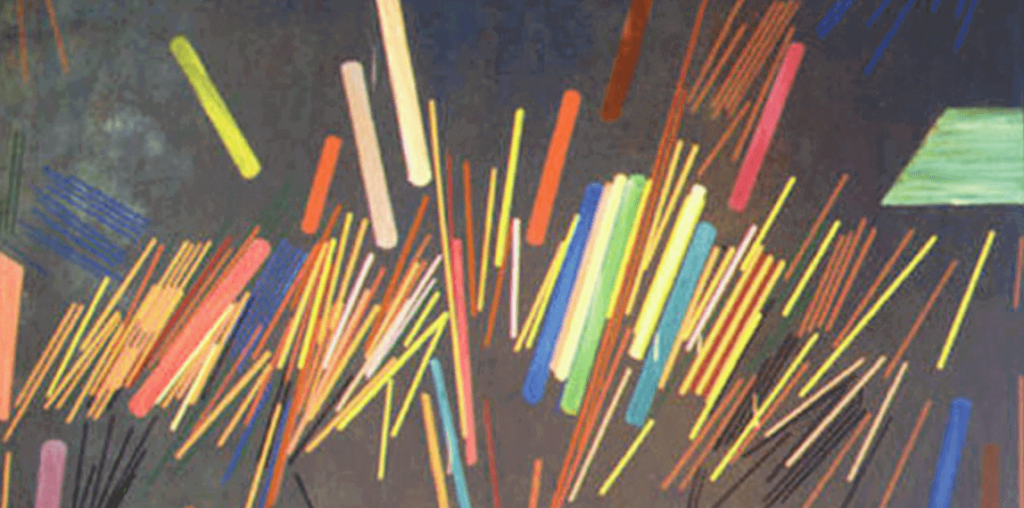
Hello ladies and gentlemen, Leo here. Today we’ll explore OperationQueue in Swift in Swift. We will learn how it can help you improve your async operations. The scope is introductory therefore we won’t going to explore every possibility with OperationQueues, the focus is the basics of the API. The main goal to achieve is how to […]
What are Lazy Variables and Why Use in Swift
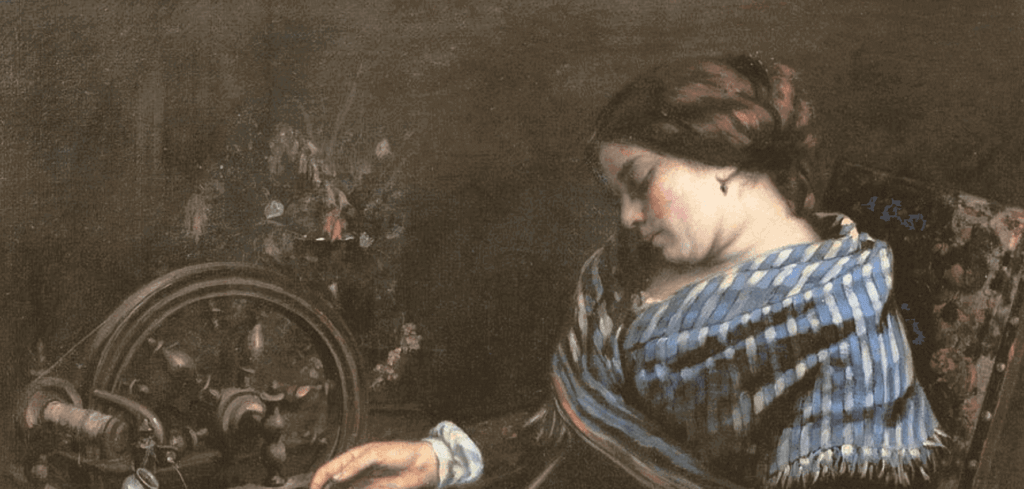
Hello ladies and gentlemen, Leo here. Today the topic is lazy variables and why it’s important for you to know. The lazy var is a special type of *var* that means the variable value isn’t calculated until we first use it. This brings to the table the flexibility of having 1000 items in memory that […]
Safe Get a Value From an Array in Swift
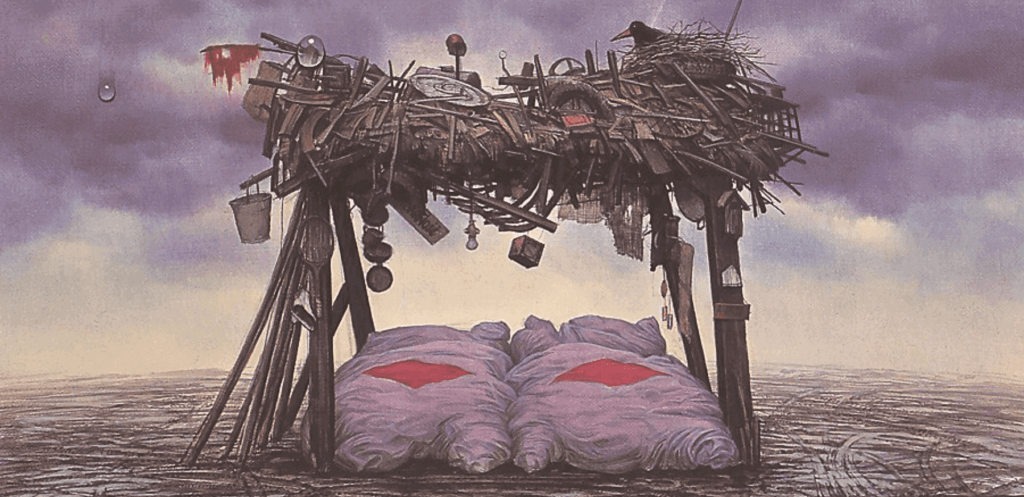
Hello people, Leo here. The topic today is how to Safe get a value from an array in Swift. Why this is important? Well, if you don’t have a mechanism to safe get values from your arrays, one day your app can crash in production because you didn’t cover all the edge cases for your […]
BFS and DFS Templates Algorithms in Swift
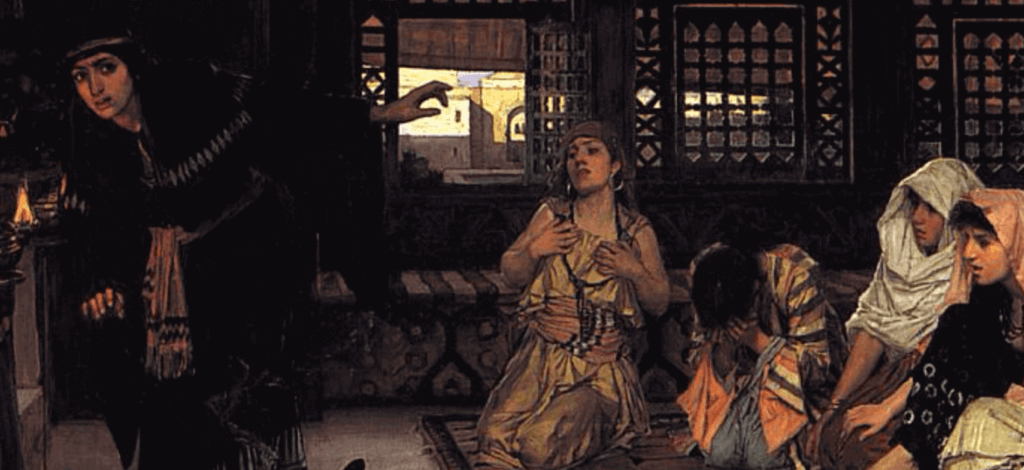
Hello everyone, Leo here. The topic today is BFS and DFS templates algorithms for problems and what is the minimum code you can have to achieve them in Swift. Today we’ll explore two very important concepts that are crucial for problem-solving that involves traversing graphs, trees, etc. Those two algorithms are the foundation of knowledge […]
UIViewController Memory Warning on Unit Tests
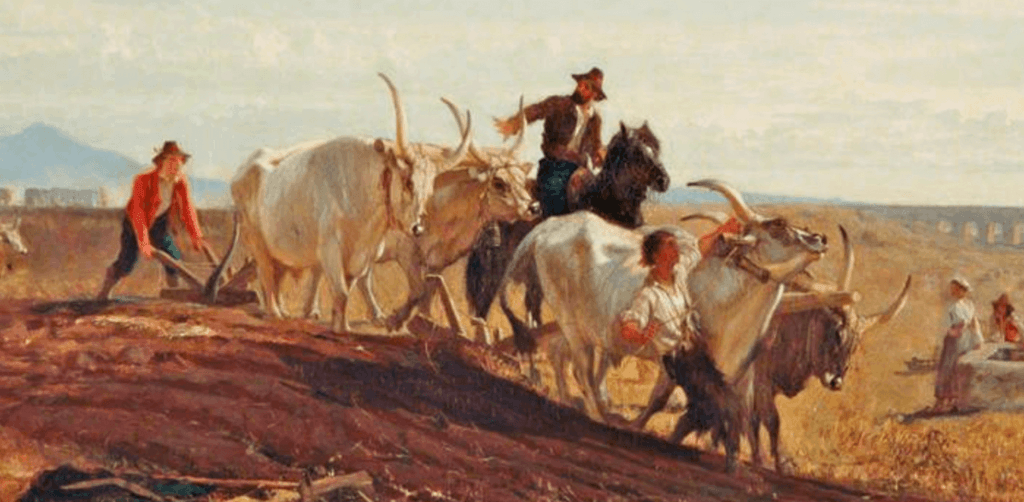
Hello brothers and sisters, Leo here. Today’s topic is how to trigger the UIViewController memory warning on your unit tests in Swift. We’ll explore some *hacks* to trigger the memory on the UIViewController. Let’s go! Problem – The UIViewController memory warning You have to test the code inside the didReceiveMemoryWarning() method from a view controller in […]